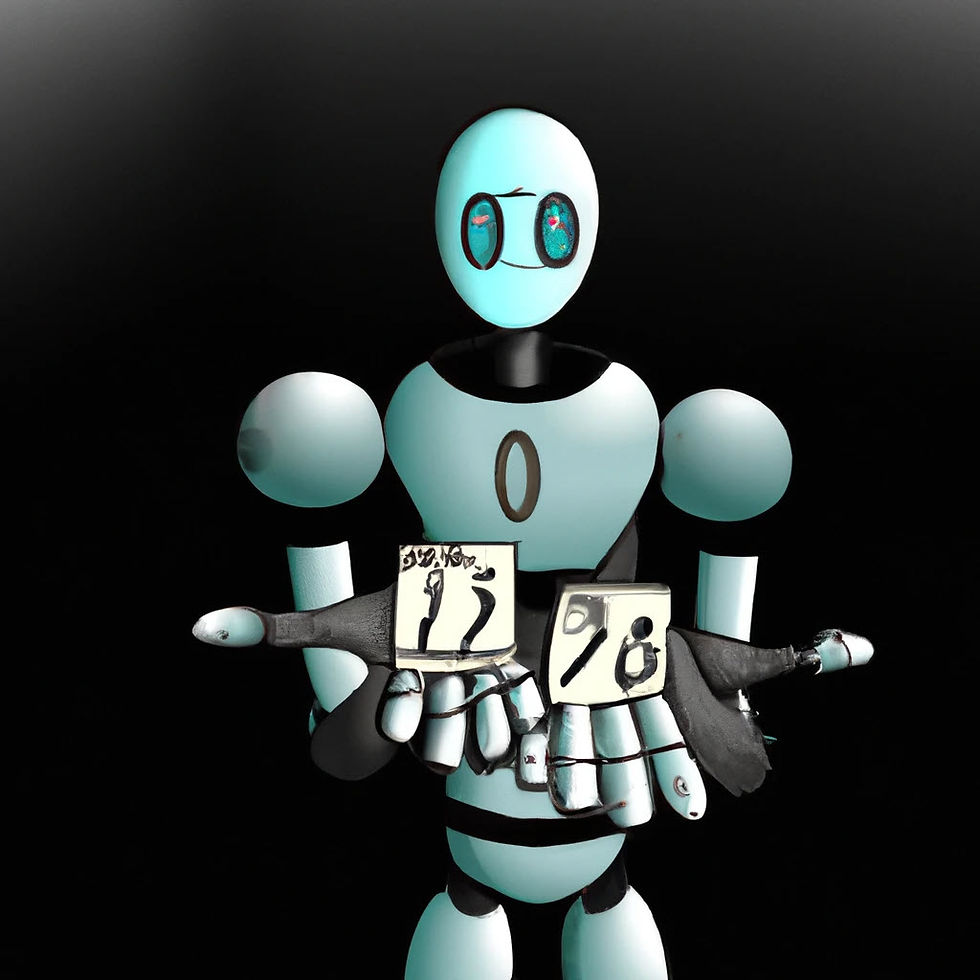
In the bustling, fast-paced world of software development, time is everything! Enter ChatGPT, a language model developed by OpenAI that's set to revolutionize your coding routine.
With a little training and tinkering, it can help automate a major part of your code review process. Think about all those style violations and sneaky bugs that slip through. ChatGPT can help catch those, making your code cleaner and your life easier!
Here's a simple guide to get you started with a proof-of-concept:
Step 1: Get Ready to Code!
Grab an API key from OpenAI, install the GPT-3 library and set up your favourite development environment.
Step 2: Training Day
Now, get ChatGPT in shape for code reviews. You do this by creating training data that shows what typical code review comments look like.
For instance, if you often forget to comment your complex code (we've all been there!), you can use examples like this:
#python
def add_numbers(a, b):
return a + b
The review comment for this could be something like, "Hey, how about adding comments to explain what your function is doing?"
Then you feed these examples to ChatGPT via the API.
Step 3: Get Connected
Once ChatGPT is all trained up, it's time to integrate it with your code review platform. GitHub, Bitbucket, whatever you're into. You could use webhooks or the platform's API to get ChatGPT into the mix every time a pull request comes in.
Step 4: Put it to the Test
You've got your system set up, and now it's time to see it in action. Try it out with some test code and see how ChatGPT does.
For instance, if you give it something like this:
#python
for i in range(10):
print(i)
ChatGPT might come back with, "Looks like 'print(i)' needs to be indented."
Let's see this in action with a bit of Python code that uses the OpenAI API for a code review:
#python
import openai
openai.api_key = 'your-api-key'
def chat_with_gpt3(prompt):
response = openai.ChatCompletion.create(
model="gpt-4.0-turbo",
messages=[
{"role": "system", "content": "You are a helpful assistant for code review."},
{"role": "user", "content": prompt}
]
)
return response['choices'][0]['message']['content']
code_sample = """
def add_numbers(a, b):
return a + b
"""
review = chat_with_gpt3(code_sample)
print(f"Code Review Comment: {review}")
Just replace 'your-api-key' with your actual OpenAI API key, and you're good to go! This script will chat with GPT-3, give it a system message defining its role as a code reviewer, then provide a code snippet for it to review. It'll then return the review comment.
Remember, while ChatGPT is pretty smart, it isn't quite ready to replace human code reviews just yet. For now, consider it for helping you catch those easy-to-miss yet crucial issues, giving you more time to focus on the fun parts of coding!
コメント